WebPack - Applying Webpack to a Real Project
A Real World Project
src:
https://github.com/StephenGrider/WebpackProject
$ git clone https://github.com/StephenGrider/WebpackProject.git
# cd WebpackProject/
# npm install
Setting Up Babel
webpack.config.js
module: {
rules: [
{
use: 'babel-loader',
test: /\.js$/,
exclude: /node_modules/
},
{
use: ['style-loader', 'css-loader'],
test: /\.css$/
}
]
}
Minimum Webpack Config
.babelrc
{
"presets": ["babel-preset-env", "react"]
}
# npm run build
Vendor Asset Caching
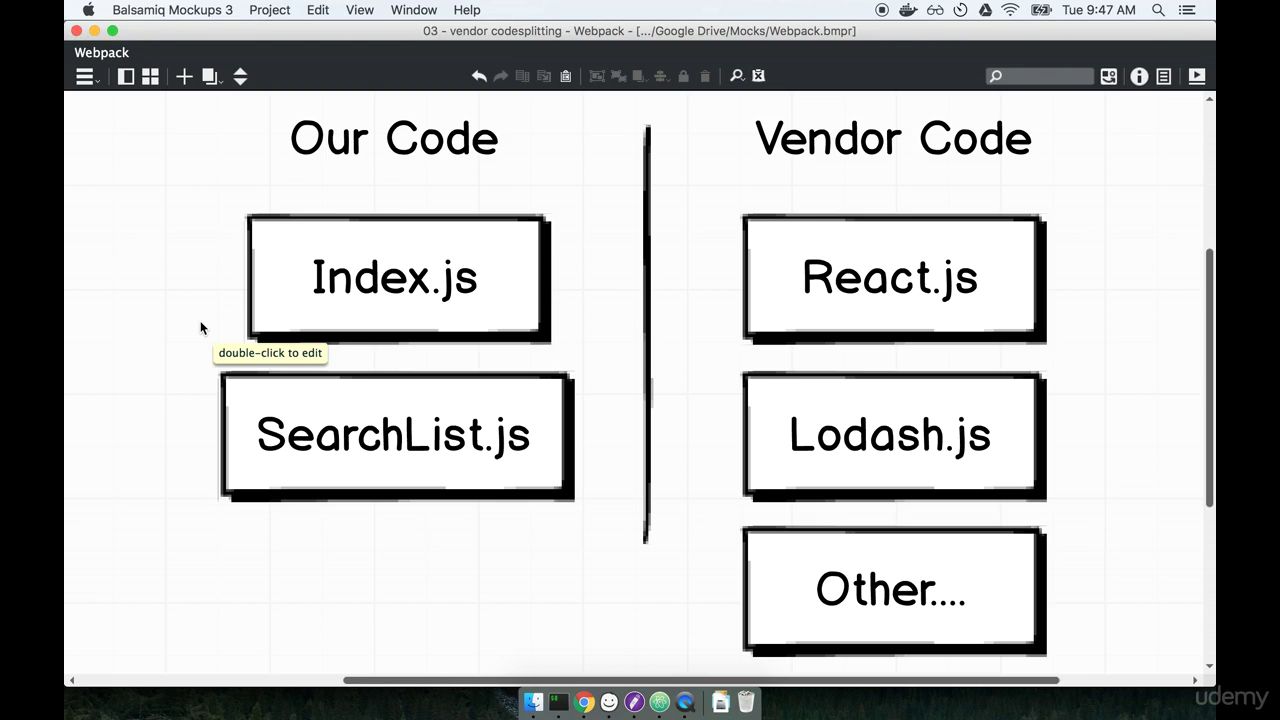
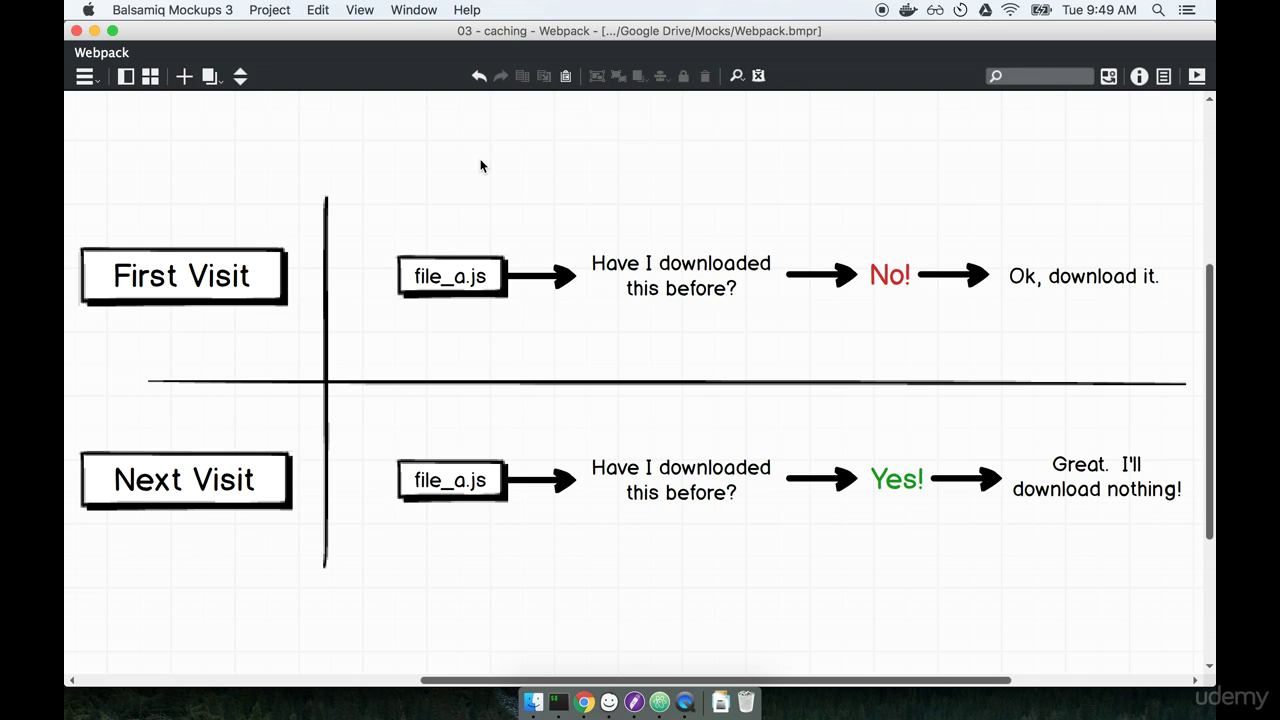
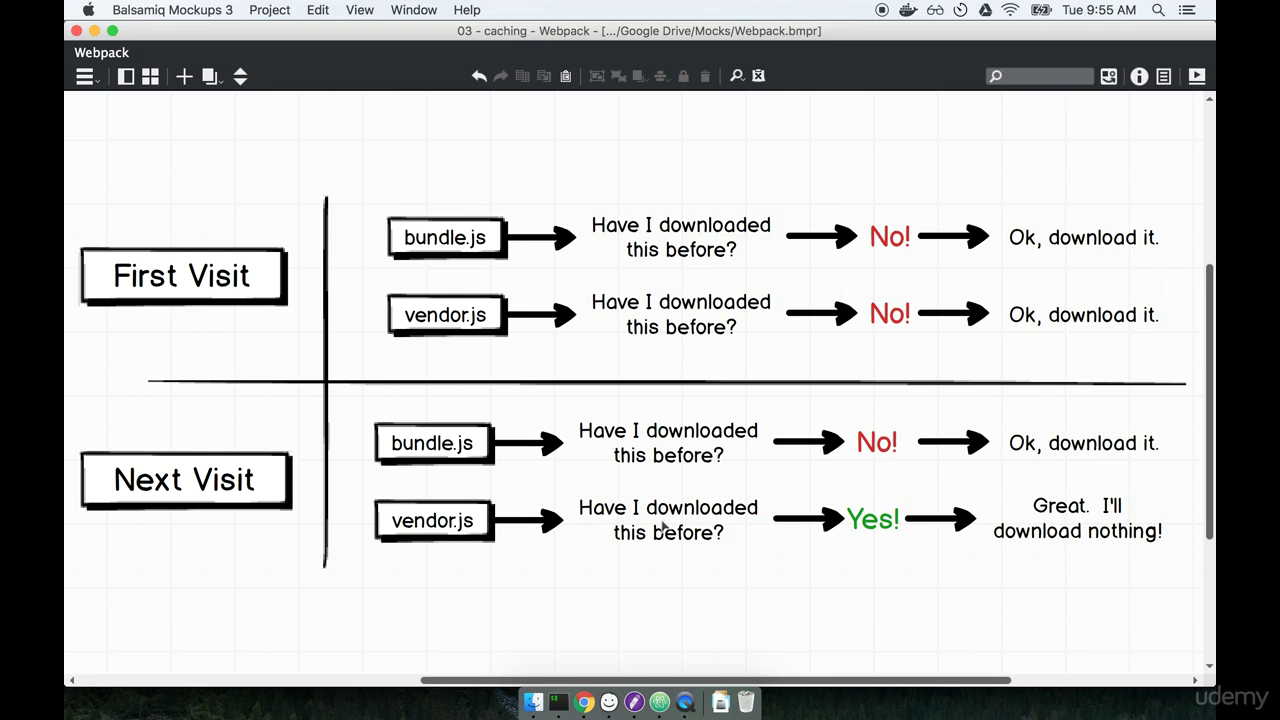
Refactoring for Vendor Splitting
webpack.config.js
const VENDOR_LIBS = [
'faker',
'lodash',
'react',
'react-dom',
'react-input-range',
'react-redux',
'react-router',
'redux',
'redux-form',
'redux-thunk'
];
module.exports = {
entry: {
bundle: './src/index.js',
vendor: VENDOR_LIBS
},
output: {
path: path.join(__dirname, 'dist'),
filename: '[name].js'
},
# npm run build
Effect of Code Splitting
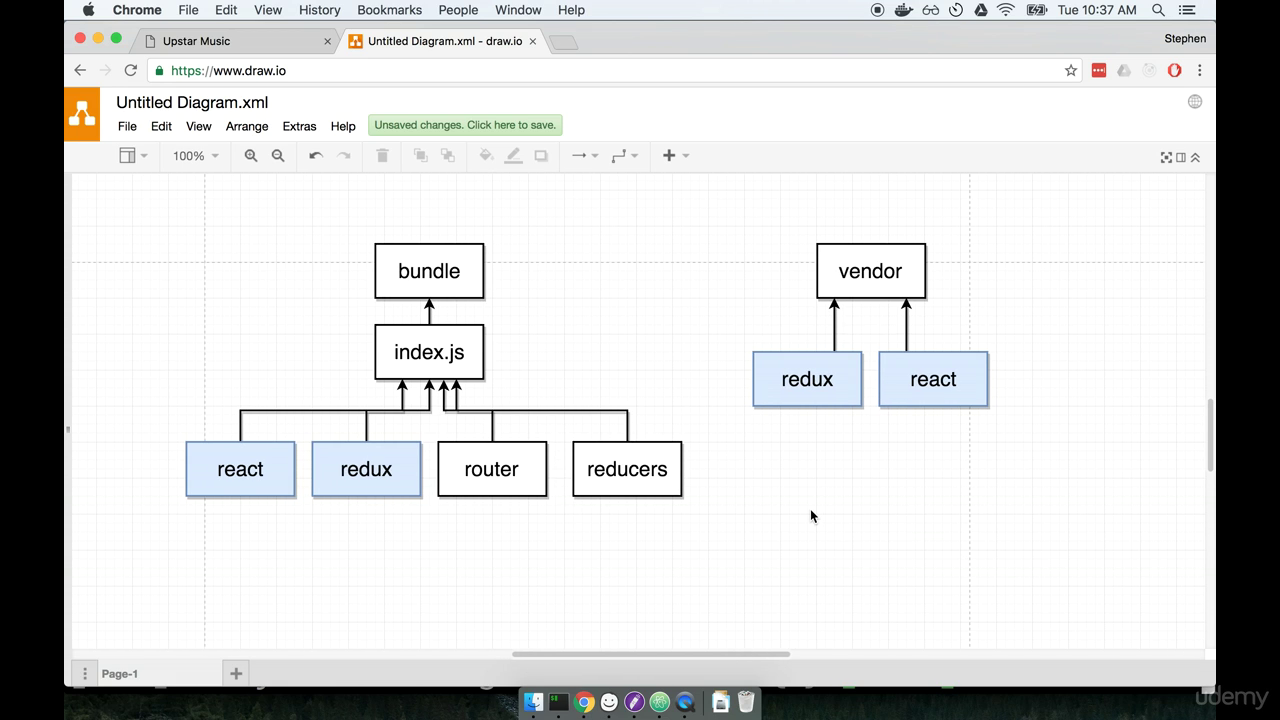
webpack.config.js
plugins: [
new webpack.optimize.CommonsChunkPlugin({
name: 'vendor'
})
]
Troubleshooting Vendor Bundles
# npm install --save-dev html-webpack-plugin
webpack.config.js
***
var HtmlWebpackPlugin = require('html-webpack-plugin');
***
new HtmlWebpackPlugin({
template: 'src/index.html'
})
Chunk Hashing for Cache Busting
webpack.config.js
***
filename: '[name].[chunkhash].js'
***
names: ['vendor', 'manifest']
Cleaning Project Files
# npm install --save-dev rimraf
package.json
"scripts": {
"clean": "rimraf dist",
"build": "npm run clean && webpack"
},
# npm run build